In making the interface for my ORPG Avatar of Destiny I came across the ugly problem of the camera clipping through the terrain. After extensive searching I could find nothing on getting it to happen so I spent some time figuring out a relatively simple method to implement it.
This is a basic tutorial on getting the camera to collide with the terrain as well as non-pathable portions of the terrain.
The most important thing is getting the camera height. This is done by some simple math:
(CameraPitch/90)*CameraDistance=CameraHeight
One thing to note that in SC2 the cameras pitch when it sinks below 90 degrees does NOT go to -1 it goes to 360 instead. So we have to account for this by subtracting 360 from it IF its over 90 degrees (I did 180 on the test map just in case).
Now that we know how to get the camera height we can do a simple trace.
CameraCollisionOptions:Action,CreateThreadReturnType:(None)ParametersPlayer=0<Integer>GrammarText:CameraCollision(Player)HintText:(None)CustomScriptCodeLocalVariablesTargetPoint=NoPoint<Point>DistanceMax=20.0<Real>DistanceChange=(DistanceMax/40.0)<Real>CameraHeight=0.0<Real>Pitch=0.0<Real>Yaw=0.0<Real>TempDistance=DistanceChange<Real>ActionsVariable-SetTargetPoint=(PositionofUnit)Variable-SetYaw=(180.0+(CurrentcamerayawofPlayer))Variable-SetPitch=(CurrentcamerapitchofPlayer)General-If(Conditions)thendo(Actions)elsedo(Actions)IfPitch>180.0ThenVariable-ModifyPitch:-360.0ElseVariable-ModifyPitch:/90.0//The 0.5 is how high off the ground the Cameras Target point is.Variable-SetCameraHeight=(0.5+(GroundheightatTargetPoint))General-While(Conditions)aretrue,do(Actions)ConditionsTempDistance<DistanceMax//The Or here causes it to collide with non-Pathable terrain but not if theres a cliff below you (note: this WILL collide with the very edge of a cliff at times)OrConditions((TargetPointoffsetbyTempDistancetowardsYawdegrees)ispassable)==true(ClifflevelofTargetPoint)>(Clifflevelof(TargetPointoffsetbyTempDistancetowardsYawdegrees))(CameraHeight+(Pitch*TempDistance))>(0.25+(Groundheightat(TargetPointoffsetbyTempDistancetowardsYawdegrees)))//The 0.25 added to the ground height is how high off the ground the camera should float (to avoid clipping into foliage etc)ActionsVariable-ModifyTempDistance:+DistanceChangeCamera-ApplycameraobjectDistance(TempDistance-DistanceChange)forplayerPlayerover0.2secondswith100%initialvelocityand0%deceleration
We have it cycle through to find if the cameras height falls below the terrain height, if it does the while loop stops and the cameras distance is set to that distance.
Looks great, highly detained and almost professional. The only gripe I have is...
Is there a reason you're avoiding the data editor? I can't see how you can make high quality spell effects using only triggers.
The main reason is the limits of how it can interact with my npc/combat system. Right now data based abilities are really hard to modify on the fly for crits misses and modifiers and the hard limit of 500k potential damage wont fit in with the model I have laid out.
My system uses integers for damage and health and I have an entire system for buffs auras etc already in place and working. Its just actually creating the spells that I have been slacking on.
I do plan to use data to store the spell descriptions icons and names however to save script space. I already have been migrating traits over and have all 250 traits already in data in the form of upgrades, though I don't use the upgrade as an upgrade just as a data storage for the components of the trait.
The other thing is that units have such a small limit as to the number of abilities they can have active on a bar at once and the key-bind system and how it works is just clunky and not intended for a more mmo-style of play. Sadly there is of course a delay when using hotkeys for my action bars though it seems clicking the buttons works pretty quickly.
If you know of a way to force a unit to cast a spell they don't have in their ability list let me know, till then I don't have much choice on not using the data editor :/
PS: also if you know of a way to get the selection circle for a spell like psi storm through triggers (even giving the unit the spell or what have you) let me know. I could really use a data driven area effect spell targeting system. I have a trigger based system but there's a delay on it which kind of sucks.
Eiviyn is a bit data-obsessed ;) Triggers are easier (at least for me) but data is more organized and it can be movied around via a mod file- something important for a project with multiple maps.
I plan to turn my script into a library and have it located in a mod as well, only map specific scripts for fights as well as monster spawn data will be in the individual maps.
As of right now I do not have a download but I do have a description.
Update 8/17/12:
Progress of rebuilding the databases is slow and grueling, but I'm working it through, if anyone who reads this wants to help with interface graphics or icons let me know. Im working on a systemon the side for better looking menus with corner images etc.
The character creation screen
The top right window will be a preview window for the character and the buttons on bottom will be replaced with class icons. The buttons under the preview window will also be changed to gender buttons once I make them. All of the components are animated and move or fade in and out.
Avatar of Destiny is an ORPG in the style of WoW/Rift I have been working on for quite some time now.
I have spent the better part of a year on this project and have thus far been mostly alone. With exception of course to the help I've received over time from the sc2mapster community on various little nibblets.
It will support (currently) up to 10 players, and will feature multiple maps or "zones" as well as dungeon and raid maps. Currently the maps all share a set of mods which contain all the art/sound/script assets so the maps themselves are relatively small.
Nearly the whole system is made in the GUI editor even health stats and spells are made this way. The data editor has some extreme limitations which I just couldn't deal with. 100k hp as a max will not work for me, nor will 500k max damage. Although I doubt any of my players will hit for 500k or more I don't like such a small number being my limit. As it stands bosses can have up to 999m hp and damage numbers can be the same.
Current Status:
Minimap with tracking
Action bars with bindable hotkeys and the ability to activate/use items or spells
Camera/Terrain collision
Full combat system- dodges, misses, criticals and deflects (parry/shield block) as well as the spell counterparts
Character stats- Basic stats and secondary stats such as haste hit crit etc.
Spell system- Is about 85% finished with only some area effect things to finish as well as implementing missiles fully.
Spells- The actual spells are only about 10% done in terms of making each of the roughly 250 I have on paper/excel spreadsheets. But my system allows for them to be easily made as I do not use the data editor at all.
Status effect system- About 75% finished just need to finish auras.
Complex Item System- Aside from the crafting menu and some socket work is mostly finished as well.
Races are fully implemented although I have only one model working right now (Teros female), though the rest wont be hard to implement. The wow models I am using are temporary until I can get models made for each race/sex combination.
Classes are also mostly done with only fully fleshing out the trait trees. I have all the traits designed on paper but only a bit more than half actually do anything.
Basics:
Characters have typical HP and MP, they also have Energy and Focus.
Energy is required by all spells and all abilities. Players have 100 and regain 10 per second. There are ways to increase the regen rate as well as the maximum for some classes or races.
Focus is a bit different. Players have 10 focus (usually) and certain maintained spells, such as auras and summons, use this focus. Summon Lesser Ent for example uses 2 focus for each Ent summoned. When a player is using all 10 focus they cannot activate any more focus using abilities until they free up enough focus.
Races:
There will be 4 races: Geijen, Elde, Teros and Zhaan.
Geijen:
Racial Bonuses: 20% increased Health, 20% reduced Mana, Awareness (see below), +1 Armor skill, +1 Shield skill
The Geijen are a race of hulking subterranean people. Geijen are known to live for hundreds of years, a few even up to over a thousand. They have white skin pale hair and are blind and physically mute. Geijen however though are telepathic and have a form of telekinetic sonar. They send out waves of psychic energy all around them. These waves then bounce back giving them the details of their surroundings as well as faint "impressions" of anything living and its general state of mind.
This telekinetic sonar gives Geijen 360 degrees of awareness and as a racial grants them the ability to deflect and dodge attacks from any angle. Geijen can "speak" through telepathy as well. Despite their fierce appearance Geijen are a gentle race and worship the "Earth God."
The Elde are a race of great intelligence and arrogance. A violent race known to start many wars, almost always losing them.
Many years ago the Elde discovered the Geijen race and "enslaved" them believing them to be nothing more than dumb brutes good for basic labor. The Geijen however though allowed this to happen as they saw great potential in the Elde, if they could be calmed. Over many decades the Geijen exerted their psychic influence to slowly quell the Eldes propensity for violence.
It was when the Silver Prince Daedelous, so named for his brilliant silver hair, came to power that the slavery of the Geijen ended and a new "Silver Age" as it was called began. The Geijen revealed their true nature to the Elde at this point, much to the shock of the Elde. There are still many of the upper class Elde who are mistrustful of the Geijen however.
Teros
Racial Bonuses: 10% increase to all stats, +1 to any weapon skill they already have 1 point in
The Teros are the peacekeepers of the land. Led by their Queen Archimedea they have many a time put the Elde in their place. Archimedea is known throughout the world for both her beauty and mystery.
Though most Teros live no more than 100 years the Queen is thousands upon thousands of years old, older than any Geijen or anything else known. As well as her seeming infinite age she holds great power, single-handedly halting an entire Elde army more than once in history- without permanently harming a single one.
Recently the Zhaan have been forced out of their woodland homes by a great shadow and have taken refuge in the Teros city of Asgarde.
The Zhaan are a race of small somewhat primitive feline-like people known for their inquisitive nature as well as their cunning and speed. Recently they have been forced to take up residence within the safety of the Teros capital city of Asgarde. Normally tribal in nature and living in small communities they have formed a council of elders and united with the Teros to try to take back their homes. Creatures of fear and shadow have been coming from the cursed lands pushing farther out than before, past the great magic barrier that has stood surrounding the area for all of history.
Classes:
There are 22 classes (currently) within 3 tiers.
The first tier is your basic Fighter Caster Healer classes. Each class has a trait tree which ends with new class selections. The fighter for example can become a Scout a Pathfinder or a Brawler. Each class has a stat focus, such as Brawler being strength and scout being agility.
However the third tier of classes also has hybrid classes. Hybrids are classes such as Beastmaster, Paladin and Wild Keeper. Hybrids may have a single or 2 focus stats such as the Paladin focusing on both wisdom and strength.
Hybrid classes are special in that they are a convergence of 2 archetypes of classes. A Cleric or a Brawler can become a Paladin, the Paladins abilities and stats will be greatly influenced by which class you had before.
Additionally each of the 12 third tier classes also has 3 "Ultimate" traits. A player may only choose one of these traits, but its effects greatly change a character.
A Wild Keeper, which is a hybrid of a Summoner and a Naturalist, is a pet based healing class summoning Ents to aid them in healing or to defend them. The Wild Keepers 3 ultimates are (names not finalized) Greater Ent, Dryad and Forest Walker. Greater Ent is actually a tanking ability as the Ent summoned is a powerful tank which the Wild Keeper will be able to "hitch a ride" on and directly control while still being able to cast their own spells from his shoulder. Dryad summons a powerful forest creature which will aid the Wild Keeper in healing with its own quite impressive heals. Lastly Forest Walker reduces the Focus cost of all of the Wild Keepers summons and increases the limit on how many Ents he may have active.
Stats:
Each base stat provides a bonus for any class. Strength provides attack power as well as a small amount of crit deflect and armor. Agility provides a movement speed increase, haste, dodge and some crit. If a class has a primary stat of strength then they gain extra attack power from it.
When a class gains their primary stat they get less of the secondary stats from it. As an example an Elementalist, whose primary stat is intelligence, gains some int- they get more elemental power from it but they get less spell critical and magic armor from it as well. Conversely when a class like an Elementalist gains strength they get a much higher boost to armor from it than a Fighter would.
Items:
Items are where I have spent a lot of my time. Right now crafting is going to be the main form of getting gear.
When crafting an item a player chooses what pattern and material to use. The pattern really only determines the "look" of an item, with the material determining how powerful it is. Players can discover new patterns though a trainer or by examining an item to learn its pattern. (not all patterns can be learned) Additionally when crafting an item a player can choose its modifier, such as "Fleeting" or "of the Warrior" which determines the basic stat bonuses the item gives.
A crafted item can have a variety of modifiers as well. A critical success during crafting makes the item "Perfect" as well as a very rare "Exquisite" which is basically a *super crit.* An item can also be crafted using extra materials and will be "Reinforced" or "Double Reinforced" depending on the amount used.
Additionally when an item has been crafted it can be further refined adding the +N prefix to the name, this process requires extra meterials and has the chance of destroying the item (from which it cannot be recovered). Lastly as the player gains combat experience so to do their items. When a player upgrades to a new item they can transfer the experience of one item to another, for a fee, and at the cost of destroying the old item.
All of the above methods increase the Quality of an item. Each level of Quality increases the items power by a large amount as well as changing the color of its border and name. White, Blue, Green, Yellow, Orange, Red and Purple are the current colors in order of power.
There is another type of item however though, Mythic. Mythic items have preset stats and special graphics as well as unique Gold colored names. Mythic items can be randomly found as well as be drops from specific bosses or quest rewards. Mythic items can be refined and gain levels, thus increasing their power.
Item Sockets:
What the above picture does not show (yet) is sockets. There will be a varying number of colored sockets on any crafted item. Depending on the pattern and quality the item will have anywhere from 0 to 6 sockets (maybe even more in the future). Each socket can have one of 7 colors Red, Green, Yellow, Blue, White, Orange and lastly Prismatic. The colors are (mostly) randomly determined at creation.
Into each socket there a a few different things which can be added: Enchanted Gemstones, Monster Souls and Runic Stones. Each of these items usually has a color which when matched to the socket color causes it to Resonate. Resonance increases the bonus that socket and all other resonating sockets gain.
Enchanted Gemstones are the basic stat boosters. Red ones increase Strength, Blue ones Intelligence and so on. Resonance simply increases these bonuses gained.
Monster Souls are extremely rare drops from monsters which provide different or unusual bonuses, usually related to the monster in some way. Boss monsters also have Souls that can drop but they are very hard to get. These souls may also grow in power based on resonance the items level or players level or some other potential source. Monster Souls can be any color (including prismatic).
Runic Stones are small stones with symbols carved into them, these stones have basic bonuses which are not as good as Enchanted Gemstones. However when Runic Stones are placed in specific sequences they "unlock" powerful set bonuses. Runic Stones can be placed in any set of slots and combined with any other type of socketable, but the first and last stone must be part of a set and there can only be one set. Each Runic Stone also can come in any of the 6 colors (as well as prismatic), so matching for both resonance and sets will not be easy but worth it.
Will that work for an actor created by another actor on its units creation?
Like When I make my Avatar unit (and there will up to 10 players with one) when its actor is created it also creates a few Actors attached to it for weapon and shoulder models. So I need send a ModelSwap command to the WeaponRight actor attached to the Actor of the Avatar unit.
I guess I'm not understanding what you're saying. :x
Okay so I have it so when I create my main unit its shoulder and weapon actors are created by the actor of the main unit.
UnitBirth.AvatarCreateWeaponRight
Now I know in order to send a message to that WeaponRight I need to create a reference (in the Content part of its creation right?) to it when its created. Then I need to use send message to (reference) of the main actor of the unit. My question is how do I set this reference during the creation of the WeaponRight actor? Anything I put in the Content section just resets the entire thing to Damage. Am I missing something?
I have been trying for hours now to get a missile to launch from weapon_right or weapon_left and to be rotated 90 degrees clockwise. Everytime the missile spawns on the ground and flies sideways. I have tried SOps on the spawned missile unit but they dont seem to have any effect. I have the attack action but none of the SOps I have tried make the missile launch from the weapon_right or weapon_left points.
A bit of necro but whatev it may help:
Another possibility, if it can be achieved is to create a foliage doodad basically and not use the normal foliage but that doodad instead which has an actor and can thus be hidden through the hide actor message.
I may do that, its really not hard to create the image dots on it, I just wanted to know if there was a better way. :/
My script is already huge (15,000 lines) and has a lot of stuff to process and while I'm sure the SC engine can handle it using non-script stuff would be better I would imagine especially when it comes to threads used.
I also didn't realize but yes your version of bringing up the minimap does in fact not use the buttons the one I found before had those ugly ass buttons for pings etc in it.
0
In making the interface for my ORPG Avatar of Destiny I came across the ugly problem of the camera clipping through the terrain. After extensive searching I could find nothing on getting it to happen so I spent some time figuring out a relatively simple method to implement it.
This is a basic tutorial on getting the camera to collide with the terrain as well as non-pathable portions of the terrain.
The most important thing is getting the camera height. This is done by some simple math:
One thing to note that in SC2 the cameras pitch when it sinks below 90 degrees does NOT go to -1 it goes to 360 instead. So we have to account for this by subtracting 360 from it IF its over 90 degrees (I did 180 on the test map just in case).
Now that we know how to get the camera height we can do a simple trace.
We have it cycle through to find if the cameras height falls below the terrain height, if it does the while loop stops and the cameras distance is set to that distance.
0
The main reason is the limits of how it can interact with my npc/combat system. Right now data based abilities are really hard to modify on the fly for crits misses and modifiers and the hard limit of 500k potential damage wont fit in with the model I have laid out.
My system uses integers for damage and health and I have an entire system for buffs auras etc already in place and working. Its just actually creating the spells that I have been slacking on.
I do plan to use data to store the spell descriptions icons and names however to save script space. I already have been migrating traits over and have all 250 traits already in data in the form of upgrades, though I don't use the upgrade as an upgrade just as a data storage for the components of the trait.
The other thing is that units have such a small limit as to the number of abilities they can have active on a bar at once and the key-bind system and how it works is just clunky and not intended for a more mmo-style of play. Sadly there is of course a delay when using hotkeys for my action bars though it seems clicking the buttons works pretty quickly.
If you know of a way to force a unit to cast a spell they don't have in their ability list let me know, till then I don't have much choice on not using the data editor :/
PS: also if you know of a way to get the selection circle for a spell like psi storm through triggers (even giving the unit the spell or what have you) let me know. I could really use a data driven area effect spell targeting system. I have a trigger based system but there's a delay on it which kind of sucks.
I plan to turn my script into a library and have it located in a mod as well, only map specific scripts for fights as well as monster spawn data will be in the individual maps.
0
As a question to mods or whatever should I have this here or in the project workplace?
0
As of right now I do not have a download but I do have a description.
Update 8/17/12:
Progress of rebuilding the databases is slow and grueling, but I'm working it through, if anyone who reads this wants to help with interface graphics or icons let me know. Im working on a systemon the side for better looking menus with corner images etc.
The character creation screen
The top right window will be a preview window for the character and the buttons on bottom will be replaced with class icons. The buttons under the preview window will also be changed to gender buttons once I make them. All of the components are animated and move or fade in and out.
Avatar of Destiny is an ORPG in the style of WoW/Rift I have been working on for quite some time now.
I have spent the better part of a year on this project and have thus far been mostly alone. With exception of course to the help I've received over time from the sc2mapster community on various little nibblets.
It will support (currently) up to 10 players, and will feature multiple maps or "zones" as well as dungeon and raid maps. Currently the maps all share a set of mods which contain all the art/sound/script assets so the maps themselves are relatively small.
Nearly the whole system is made in the GUI editor even health stats and spells are made this way. The data editor has some extreme limitations which I just couldn't deal with. 100k hp as a max will not work for me, nor will 500k max damage. Although I doubt any of my players will hit for 500k or more I don't like such a small number being my limit. As it stands bosses can have up to 999m hp and damage numbers can be the same.
Current Status:
Basics:
Characters have typical HP and MP, they also have Energy and Focus.
Energy is required by all spells and all abilities. Players have 100 and regain 10 per second. There are ways to increase the regen rate as well as the maximum for some classes or races.
Focus is a bit different. Players have 10 focus (usually) and certain maintained spells, such as auras and summons, use this focus. Summon Lesser Ent for example uses 2 focus for each Ent summoned. When a player is using all 10 focus they cannot activate any more focus using abilities until they free up enough focus.
Races:
There will be 4 races: Geijen, Elde, Teros and Zhaan.
Geijen:
Racial Bonuses: 20% increased Health, 20% reduced Mana, Awareness (see below), +1 Armor skill, +1 Shield skill
The Geijen are a race of hulking subterranean people. Geijen are known to live for hundreds of years, a few even up to over a thousand. They have white skin pale hair and are blind and physically mute. Geijen however though are telepathic and have a form of telekinetic sonar. They send out waves of psychic energy all around them. These waves then bounce back giving them the details of their surroundings as well as faint "impressions" of anything living and its general state of mind.
This telekinetic sonar gives Geijen 360 degrees of awareness and as a racial grants them the ability to deflect and dodge attacks from any angle. Geijen can "speak" through telepathy as well. Despite their fierce appearance Geijen are a gentle race and worship the "Earth God."
Elde
Racial Bonuses: 20% increased Mana, +2 Dual Wield skill, +10 Spell Haste
The Elde are a race of great intelligence and arrogance. A violent race known to start many wars, almost always losing them.
Many years ago the Elde discovered the Geijen race and "enslaved" them believing them to be nothing more than dumb brutes good for basic labor. The Geijen however though allowed this to happen as they saw great potential in the Elde, if they could be calmed. Over many decades the Geijen exerted their psychic influence to slowly quell the Eldes propensity for violence.
It was when the Silver Prince Daedelous, so named for his brilliant silver hair, came to power that the slavery of the Geijen ended and a new "Silver Age" as it was called began. The Geijen revealed their true nature to the Elde at this point, much to the shock of the Elde. There are still many of the upper class Elde who are mistrustful of the Geijen however.
Teros
Racial Bonuses: 10% increase to all stats, +1 to any weapon skill they already have 1 point in
The Teros are the peacekeepers of the land. Led by their Queen Archimedea they have many a time put the Elde in their place. Archimedea is known throughout the world for both her beauty and mystery.
Though most Teros live no more than 100 years the Queen is thousands upon thousands of years old, older than any Geijen or anything else known. As well as her seeming infinite age she holds great power, single-handedly halting an entire Elde army more than once in history- without permanently harming a single one.
Recently the Zhaan have been forced out of their woodland homes by a great shadow and have taken refuge in the Teros city of Asgarde.
Zhaan
Racial Bonuses: +2 Focus, +20 Energy, +10 movement speed, +10 Haste
The Zhaan are a race of small somewhat primitive feline-like people known for their inquisitive nature as well as their cunning and speed. Recently they have been forced to take up residence within the safety of the Teros capital city of Asgarde. Normally tribal in nature and living in small communities they have formed a council of elders and united with the Teros to try to take back their homes. Creatures of fear and shadow have been coming from the cursed lands pushing farther out than before, past the great magic barrier that has stood surrounding the area for all of history.
Classes:
The first tier is your basic Fighter Caster Healer classes. Each class has a trait tree which ends with new class selections. The fighter for example can become a Scout a Pathfinder or a Brawler. Each class has a stat focus, such as Brawler being strength and scout being agility.
However the third tier of classes also has hybrid classes. Hybrids are classes such as Beastmaster, Paladin and Wild Keeper. Hybrids may have a single or 2 focus stats such as the Paladin focusing on both wisdom and strength.
Hybrid classes are special in that they are a convergence of 2 archetypes of classes. A Cleric or a Brawler can become a Paladin, the Paladins abilities and stats will be greatly influenced by which class you had before.
Additionally each of the 12 third tier classes also has 3 "Ultimate" traits. A player may only choose one of these traits, but its effects greatly change a character.
A Wild Keeper, which is a hybrid of a Summoner and a Naturalist, is a pet based healing class summoning Ents to aid them in healing or to defend them. The Wild Keepers 3 ultimates are (names not finalized) Greater Ent, Dryad and Forest Walker. Greater Ent is actually a tanking ability as the Ent summoned is a powerful tank which the Wild Keeper will be able to "hitch a ride" on and directly control while still being able to cast their own spells from his shoulder. Dryad summons a powerful forest creature which will aid the Wild Keeper in healing with its own quite impressive heals. Lastly Forest Walker reduces the Focus cost of all of the Wild Keepers summons and increases the limit on how many Ents he may have active.
Stats:
Each base stat provides a bonus for any class. Strength provides attack power as well as a small amount of crit deflect and armor. Agility provides a movement speed increase, haste, dodge and some crit. If a class has a primary stat of strength then they gain extra attack power from it.
When a class gains their primary stat they get less of the secondary stats from it. As an example an Elementalist, whose primary stat is intelligence, gains some int- they get more elemental power from it but they get less spell critical and magic armor from it as well. Conversely when a class like an Elementalist gains strength they get a much higher boost to armor from it than a Fighter would.
Items:
Items are where I have spent a lot of my time. Right now crafting is going to be the main form of getting gear.
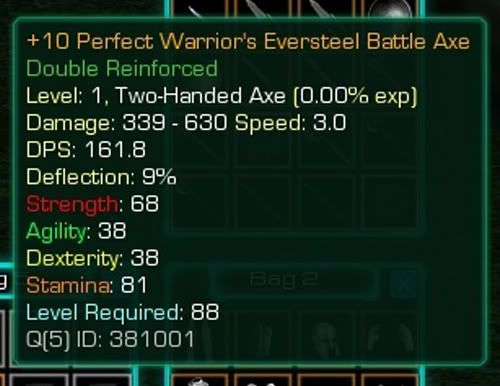
When crafting an item a player chooses what pattern and material to use. The pattern really only determines the "look" of an item, with the material determining how powerful it is. Players can discover new patterns though a trainer or by examining an item to learn its pattern. (not all patterns can be learned) Additionally when crafting an item a player can choose its modifier, such as "Fleeting" or "of the Warrior" which determines the basic stat bonuses the item gives.
A crafted item can have a variety of modifiers as well. A critical success during crafting makes the item "Perfect" as well as a very rare "Exquisite" which is basically a *super crit.* An item can also be crafted using extra materials and will be "Reinforced" or "Double Reinforced" depending on the amount used.
Additionally when an item has been crafted it can be further refined adding the +N prefix to the name, this process requires extra meterials and has the chance of destroying the item (from which it cannot be recovered). Lastly as the player gains combat experience so to do their items. When a player upgrades to a new item they can transfer the experience of one item to another, for a fee, and at the cost of destroying the old item.
All of the above methods increase the Quality of an item. Each level of Quality increases the items power by a large amount as well as changing the color of its border and name. White, Blue, Green, Yellow, Orange, Red and Purple are the current colors in order of power.
There is another type of item however though, Mythic. Mythic items have preset stats and special graphics as well as unique Gold colored names. Mythic items can be randomly found as well as be drops from specific bosses or quest rewards. Mythic items can be refined and gain levels, thus increasing their power.
Item Sockets:
What the above picture does not show (yet) is sockets. There will be a varying number of colored sockets on any crafted item. Depending on the pattern and quality the item will have anywhere from 0 to 6 sockets (maybe even more in the future). Each socket can have one of 7 colors Red, Green, Yellow, Blue, White, Orange and lastly Prismatic. The colors are (mostly) randomly determined at creation.
Into each socket there a a few different things which can be added: Enchanted Gemstones, Monster Souls and Runic Stones. Each of these items usually has a color which when matched to the socket color causes it to Resonate. Resonance increases the bonus that socket and all other resonating sockets gain.
Enchanted Gemstones are the basic stat boosters. Red ones increase Strength, Blue ones Intelligence and so on. Resonance simply increases these bonuses gained.
Monster Souls are extremely rare drops from monsters which provide different or unusual bonuses, usually related to the monster in some way. Boss monsters also have Souls that can drop but they are very hard to get. These souls may also grow in power based on resonance the items level or players level or some other potential source. Monster Souls can be any color (including prismatic).
Runic Stones are small stones with symbols carved into them, these stones have basic bonuses which are not as good as Enchanted Gemstones. However when Runic Stones are placed in specific sequences they "unlock" powerful set bonuses. Runic Stones can be placed in any set of slots and combined with any other type of socketable, but the first and last stone must be part of a set and there can only be one set. Each Runic Stone also can come in any of the 6 colors (as well as prismatic), so matching for both resonance and sets will not be easy but worth it.
0
@Kueken531: Go
Yeah for me too.
0
Title pretty much sums it up, I need to be able to change which attach point an actor is attached to via a message.
Is this even possible?
0
@Kueken531: Go
Yep, thank you it works perfect for weapon switching thanks. Now I just have to figure out why the polearm model keeps crashing sc2 ><.
0
@Kueken531: Go
Will that work for an actor created by another actor on its units creation?
Like When I make my Avatar unit (and there will up to 10 players with one) when its actor is created it also creates a few Actors attached to it for weapon and shoulder models. So I need send a ModelSwap command to the WeaponRight actor attached to the Actor of the Avatar unit.
I guess I'm not understanding what you're saying. :x
Edit:
OOOOH I got it now I do a
0
Okay so I have it so when I create my main unit its shoulder and weapon actors are created by the actor of the main unit.
Now I know in order to send a message to that WeaponRight I need to create a reference (in the Content part of its creation right?) to it when its created. Then I need to use send message to (reference) of the main actor of the unit. My question is how do I set this reference during the creation of the WeaponRight actor? Anything I put in the Content section just resets the entire thing to Damage. Am I missing something?
0
Where could i find info on making site actors? I haven't seen anything yet in my searching (maybe I'm looking in the wrong places ><)
0
http://forums.sc2mapster.com/resources/tutorials/19371-tutorial-trigger-data-show-hide-destroy-any-given-actor/
0
I have been trying for hours now to get a missile to launch from weapon_right or weapon_left and to be rotated 90 degrees clockwise. Everytime the missile spawns on the ground and flies sideways. I have tried SOps on the spawned missile unit but they dont seem to have any effect. I have the attack action but none of the SOps I have tried make the missile launch from the weapon_right or weapon_left points.
Anyone have any ideas?
0
@Builder_Bob: Go
A bit of necro but whatev it may help: Another possibility, if it can be achieved is to create a foliage doodad basically and not use the normal foliage but that doodad instead which has an actor and can thus be hidden through the hide actor message.
0
@gamfvr: Go
Possibly but I would like to implement a zoom function. And It may not scale the dots up to it.
Edit: You can't change the minimap.
0
@gamfvr: Go
I may do that, its really not hard to create the image dots on it, I just wanted to know if there was a better way. :/
My script is already huge (15,000 lines) and has a lot of stuff to process and while I'm sure the SC engine can handle it using non-script stuff would be better I would imagine especially when it comes to threads used.
I also didn't realize but yes your version of bringing up the minimap does in fact not use the buttons the one I found before had those ugly ass buttons for pings etc in it.